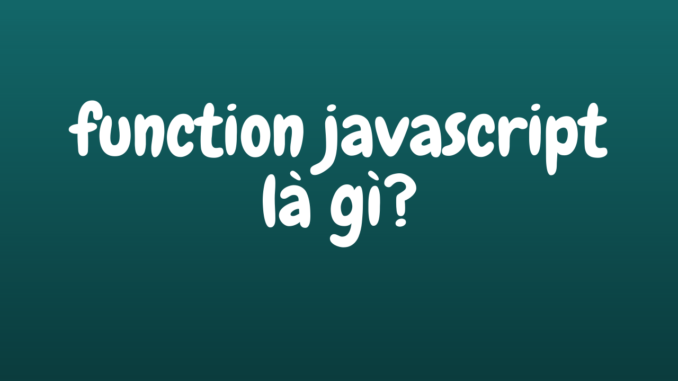
1. Function javascript là gì?
Các kiểu dữ liệu trong javascript
- Functions là các đối tượng lớp đầu tiên trong JS, giống như bất kỳ đối tượng khác
- Chúng có thể được lưu trữ trong các biến, được truyền vào các function khác dưới dạng đối số và được xây dựng tại thời điểm chạy
- Chúng có các thuộc tính (như các đối tượng)
- Chúng có thể được lồng trong các function khác
Tuyển lập trình viên PHP lương upto $2000
Tuyển lập trình viên .Net lương upto $1800
function sayHi(name) {
console.log(“Hello, ” + name);
}
sayHi(“khoibv”); // “Hello, khoibv”
- Javascript hỗ trợ function statement(câu lệnh function) và function expression(biểu thức function)
Dưới là function giống hệt nhau nhưng cách viết khác thôi.
-
- function foo(arg) { … } (function statement)
- var foo = function(arg) { … } (function expression)
- arguments` là một biến cục bộ (array) có sẵn trong mọi function. Nó chứa tất cả tham số truyền tới function
function say() {
console.log(arguments[0] + ‘, ‘ + arguments[1])
}
say(‘Hello’, ‘world’) // Hello, world
function say không có tham số, nhưng chúng ta có thể truyền và truy cập tham số bằng cách sử dụng biến arguments
- Javascript không có định nghĩa `class`, chúng ta không thể thấy từ khóa `class` trong javascript nhưng chúng ta có thể sử dụng function để khai báo một class
// Declare class Animation with 1 field `name`
function Animation(name) {
this.name = name;
}
// Declare method to start animation
Animation.prototype.start = function() {
// In this method, this.name is the value of an instance of Animation class
console.log(“Start animation ” + this.name);
};
// Declare method to stop
Animation.prototype.stop = function() {
console.log(“Stop animation ” + this.name);
};
// Test it
let instance = new Animation(“FadeOut”);
instance.start();// Start animation FadeOut
instance.stop(); // Stop animation FadeOut
- Chúng ta có thể tạo một anonymous function(function ẩn danh, không cần tên) và có thể chạy nó ngay lập tức.
- It named IIFE (Immediately Invoked Function Expression). Tạm dịch là biểu thức function được gọi ngay lập tức
Ví dụ 1:
(function(foo, bar) {
document.write(foo * bar);
})(10, 2); // 20 Cặp dấu ngoặc đơn ở cuối khai báo thực thi function ngay lập tức
Ví dụ 2: trong jquery-ui source
(function(factory) {
if (typeof define === “function” && define.amd) {
// AMD. Register as an anonymous module.
define([“jquery”, “./version”], factory);
} else {
// Browser globals
factory(jQuery);
}
})(function($) {
// jquery-ui processing
});
Ví dụ 3: Google Analytics on vnexpress.net
(function(i, s, o, g, r, a, m) {
i[“GoogleAnalyticsObject”] = r;
(i[r] =
i[r] ||
function() {
(i[r].q = i[r].q || []).push(arguments);
}),
(i[r].l = 1 * new Date());
(a = s.createElement(o)), (m = s.getElementsByTagName(o)[0]);
a.async = 1;
a.src = g;
m.parentNode.insertBefore(a, m);
})(
window,
document,
“script”,
“https://www.google-analytics.com/analytics.js”,
“ga”
);
- ES6 có ngữ pháp mới: arrow function(function mũi tên)
Nó giống như biểu thức lambda trong Java và C#
Ví dụ 1:
// normal function
function sayHello(name) {
var upperFirstLetter = name[0].toUpperCase() + name.substring(1);
console.log(“Hello ” + upperFirstLetter);
}
sayHello(“foo”); // Hello Foo
// arrow function
const sayHello2 = (name) => {
var upperFirstLetter = name[0].toUpperCase() + name.substring(1);
console.log(“Hello ” + upperFirstLetter);
};
sayHello2(“bar”); // Hello Bar
Format of arrow function:
(argument1, argument2, Hello Bar… argumentN) => {
// function body
}
Ví dụ 2:
const words = [‘hello’, ‘WORLD’, ‘Whatever’];
const downcasedWords = words.map(word => word.toLowerCase()); // hello, world, whatever
Leave a Reply